This tutorial is based on Chrome Extension Manifest Version 2.
Let's Begin.
Before we even touch a line of code, we need to setup our development environment.
Navigate to https://console.firebase.google.com/ and login.
Click 'Create Project' and name it.
If you need the 'Analytics' functionality, choose so.
Once the project is created, create a new app by clicking on the Web icon.
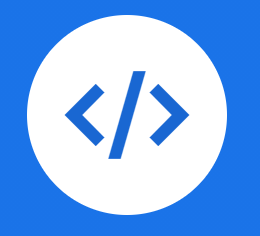
Name the app.
If you need the Firebase Hosting functionality, choose so.
Navigate back to the developer console and click on the 'Authentication' card. Click on 'Sign-in method' in the navigation bar.
Here is where you choose which sign-in options you'll be giving the user.
For this tutorial, we'll use Google and GitHub.
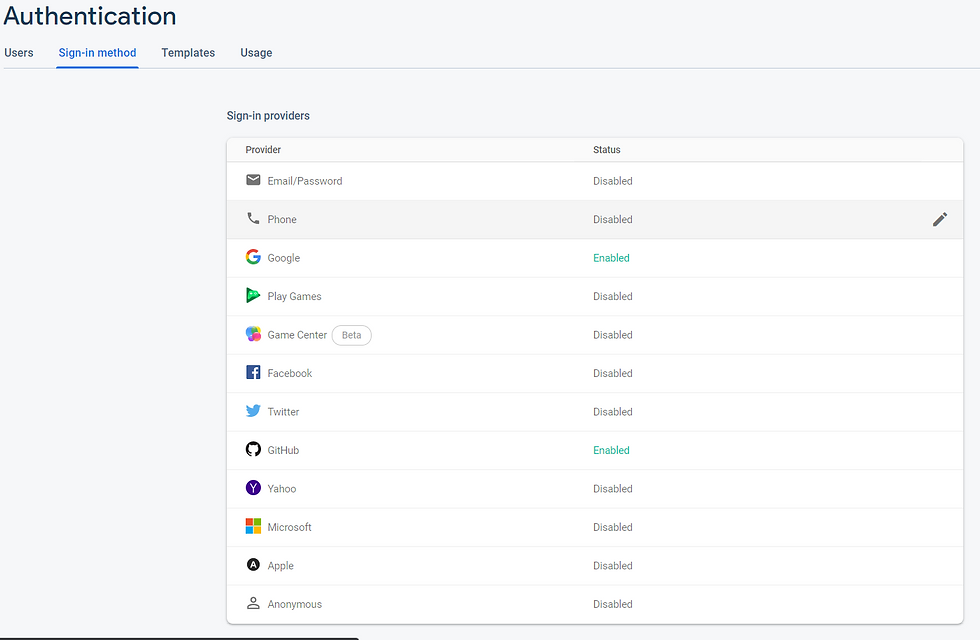
Click on the Google option, enable it, provide a Support email' and save.
Click on the GitHub option and enable it.
Copy the 'callback URL' from this page.
We need to retrieve a 'Client ID' and 'Client secret' from GitHub's developer "console".
Navigate to GitHub, click on your user icon and choose Settings.
Click on Developer settings > OAuth Apps > Create new application.
Name the application whatever you like.
The 'Homepage URL' can be anything you want.
The 'Authorization callback URL' is the 'callback URL' provided by Firebase.
Click 'Register application'.
Copy the 'Client ID' and 'Client secret' and paste these into your Firebase GitHub option.
Scroll down to 'Add domain'; we need to whitelist our Chrome extension.
Add the domain, "chrome-extension://", where is your Chrome extension id.
This can be found @ chrome://extensions/ in your browser.
Scroll down to 'Multiple accounts per email address'.
If you want, enable this ability.
We can now start with some actual coding.
Let's do some web development work before we get to actual Chrome Extension work.
We'll need three pages.
A 'popup.html' which we'll use to give the user 'sign in' options.
A 'welcome.html' which we'll show to inform the user they've been successfully logged in.
A 'main.html' which we'll use to give the user the option to 'sign out'.
Note: Take note of all of the 'scripts' attached to our HTML files. We'll fill those out as we go.
Now that we have the Web Dev portion out of the way, let's take a look at our 'manifest.json'.
Note: The "content_security_policy" is essential for this app. It allows Firebase to download its necessary files.
Let's do some actual Chrome Extension programming.
We'll start by coding the basic skeletal logic flow of our app.
In the 'main-script.js' script, when the user clicks on the diamond, we'll send a message to the 'background.js' script asking to "sign_out".
If we get a "success" from the 'background.js' we'll change the page to the 'popup.html' page.
// main-script.js
document.querySelector('#sign_out').addEventListener('click', () => {
chrome.runtime.sendMessage({ message: 'sign_out' },
function (response) {
if (response.message === 'success') {
window.location.replace('./popup.html');
}
}
});
});
In the 'popup-init.js' script, we'll check whether or not the user is currently signed in.
If they are, we'll show them the 'main.html' page; if they are not, we'll show them the 'popup.html' page.
// popup-init.js
function init() {
chrome.runtime.sendMessage({ message: 'is_user_signed_in' },
function (response) {
if (response.message === 'success' && response.payload) {
window.location.replace('./main.html');
}
}
});
}
init();
The 'popup-script.js' is where we'll do most of our coding.
Before that however, we need to download the Firebase SDKs. So in the 'popup.html', we'll include some scripts and links in the head.
// popup.html
<script src="https://www.gstatic.com/firebasejs/7.19.1/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.19.1/firebase-auth.js"></script>
<script src="https://cdn.firebase.com/libs/firebaseui/3.5.2/firebaseui.js"></script>
<link type="text/css" rel="stylesheet" href="https://cdn.firebase.com/libs/firebaseui/3.5.2/firebaseui.css" />
Let's get to the popup-script.js.
We'll be copying and pasting a lot of code from
Navigate to the settings of your Firebase Web App.
Scroll down until you find the 'Firebase SDK snippet'.
We just need the 'Config'.
Copy and paste that into your 'popup-script.js' and make sure to initialize.
// popup-script.js
const firebaseConfig = {
apiKey: "",
authDomain: "",
databaseURL: "",
projectId: "",
storageBucket: "",
messagingSenderId: "",
appId: ""
};
firebase.initializeApp(firebaseConfig);
Next, we'll:
initialize the ‘Firebase Auth UI’
setup the options for the UI
then start the UI when the user clicks the ‘Sign In’ button
Note:
In the 'signInSuccessWithAuthResult: function (authResult, redirectUrl)' of our 'uiConfig'’, we send a message to 'background.js' script informing our extension that the user has successfully signed in.
In the 'uiShown: function()' we make sure to hide the 'Sign In' button.
We don't need a value for 'signInSuccessUrl' since Chrome doesn't allow us to redirect back to it.
There you have it. Firebase Authentication brought to Google Chrome Extensions.

Comments